
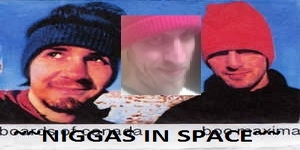
User Controls
Help a brother create a script/program
-
2016-01-25 at 1:01 AM UTCIn my area, the most popular internet provider seems to have these public hot spots everywhere. I'm not sure if the are part of legit customers routers that they 'maybe get' when signing up for the service, but these all have a captive portal that allows people to sign up for a complimentary session that'll last for one hour. After the hour is up, you can't sign up for another session until 24 hours has passed, and you can only get like two sessions in a month.
The good part, is that these keep track of the users via MAC address, and there are a bunch very close to me(like ten or so). I can spoof my MAC and connect over and over again, giving me 100% free internet, although I wont do banking or anything like that on the connection.
Anyways, I'd like to create a small script that will spoof my MAC address every 55 minutes, reconnect to the access point, and then sign up for a session. I also wouldn't mind some type of interface that would show how much time I have left on the current session, just in case I want to download a large file(to prevent an interruption).
Edit: I'm running Ubuntu 14.04
Any links would be appreciated. -
2016-01-25 at 1:12 AM UTC
-
2016-01-25 at 3:58 AM UTCSadly you don't even python because it just so happens there's a script that spoofs MAC already. https://github.com/feross/SpoofMAC
Basically what i would do is get rid of option parsing and substitute a set of values that i know would apply to my system/configuration and randomize MAC selection by default which as it happens can already be done in the script i posted above, then i'd just put it on a timer.
import time
def timer():
while True:
try:
time.sleep(3300)
spoof_mac()
except KeyboardInterrupt:
print "User aborted the script."
def spoof_mac():
# MAC spoof code here.
timer()
spoof_mac()
Or something like that. I'm pretty sure you could do it in a more elegant way than this, but this is just from the top of my head. As to the GUI you could use Tkinter a quick google search showed me this as far as having a countdown clock is concerned.
try:
# Python2
import Tkinter as tk
except ImportError:
# Python3
import tkinter as tk
import time
def count_down():
# start with 2 minutes --> 120 seconds
for t in range(120, -1, -1):
# format as 2 digit integers, fills with zero to the left
# divmod() gives minutes, seconds
sf = "{:02d}:{:02d}".format(*divmod(t, 60))
#print(sf) # test
time_str.set(sf)
root.update()
# delay one second
time.sleep(1)
# create root/main window
root = tk.Tk()
time_str = tk.StringVar()
# create the time display label, give it a large font
# label auto-adjusts to the font
label_font = ('helvetica', 40)
tk.Label(root, textvariable=time_str, font=label_font, bg='white',
fg='blue', relief='raised', bd=3).pack(fill='x', padx=5, pady=5)
# create start and stop buttons
# pack() positions the buttons below the label
tk.Button(root, text='Count Start', command=count_down).pack()
# stop simply exits root window
tk.Button(root, text='Count Stop', command=root.destroy).pack()
# start the GUI event loop
root.mainloop()
Substitute appropriate values for the time you require obviously. I hope that helps. -
2016-01-25 at 4:09 AM UTCOR we could also do this in bash and just set it as a cronjob to fire every 55 minutes. This is a better idea nigga in fact just have bash select a mac randomly from a list of strings, then it's like 3 lines of code to change your mac to that value and the cronjob will do all the looping and timing for you.
-
2016-01-25 at 4:16 AM UTCI've looked into Python a little but not much. I have compiler on my RaspPi. I'll look into it a bit more, although C is something I'd prefer.
-
2016-01-25 at 4:25 AM UTC
I've looked into Python a little but not much. I have compiler on my RaspPi. I'll look into it a bit more, although C is something I'd prefer.
Yeah, or just use bash like i mentioned above. That would be the easiest option. -
2016-01-25 at 6:49 AM UTC
Yeah, or just use bash like i mentioned above. That would be the easiest option.
I most likely will start there, but I'd like to know how much time the connect has left. Either way, this is a good project for me and at a good time. I'm ready to move on from Java/Android(even though I like it a lot) and learn more of the lower level stuff. I'll post post what I come up with here... -
2016-01-25 at 7:09 AM UTCI know it's a little late but +1 for the bash idea - seems the simplest one.
Also, ubuntu has this "notify-send" command that will make a nice notification appear in the upper right corner of your screen. You can add it to the bash script so that you get notifications this way ie. "10 minutes remaining". -
2016-01-25 at 1:10 PM UTC
I know it's a little late but +1 for the bash idea - seems the simplest one.
Also, ubuntu has this "notify-send" command that will make a nice notification appear in the upper right corner of your screen. You can add it to the bash script so that you get notifications this way ie. "10 minutes remaining".
Oh yeah, we got notify send as well. Awesome, niggas underestimate the power of Bash. I fucking love Bash.I'll post post what I come up with here…
I'd be very interested to see the results of your efforts. -
2016-01-25 at 3:40 PM UTCThis is awesome.
I've never actually written a bash script before. If I have some spare time this week I'll look into this. -
2016-01-27 at 8:36 AM UTCHere's what I have so far. I can spoof my MAC to a random address with this and I can return it back to the original(but it does require me to enter it in before hand).
#!/bin/bash
hexchars="0123456789ABCDEF"
rndMAC=
origMAC=00:00:00:11:23:11
function help() {
cat << EOF
Usage: $0 [args]
-h, --help - Print this help and exit
-r, --run - Spoof MAC every 55 minutes and connect/login to hotspot
-s, --spoof - Spoof MAC address to a randomly generated address
-u, --unspoof - Return spoofed MAC address to original MAC address
EOF
}
function genMac() {
end=$( for i in {1..10} ; do echo -n ${hexchars:$(( $RANDOM % 16 )):1} ; done | sed -e 's/\(..\)/:\1/g' )
rndMAC=00$end
}
function changeMac() {
MAC="$1"
service network-manager stop
ifconfig wlan0 down
ifconfig wlan0 hw ether $MAC
ifconfig wlan0 up
service network-manager start
}
function run() {
genMac
changeMac $rndMAC
#should automaticall connect due to previous connections
#login is next
#wait 55 minutes
run
echo "TODO"
}
if [ $UID -gt 0 ] ;then
die "RUN AS ROOT NIGGA"
fi
while [ $# -gt 0 ]
do
case "$1" in
"-h"|"--help")
help
die
;;
"-r"|"--run")
run
;;
"-s"|"--spoof")
genMac
changeMac $rndMAC
;;
"-u"|"--unspoof")
changeIface $origMAC
;;
*)
help
die
;;
esac
shift
done
I'll use BurpSuite when signing up for a new session and see what kind of HTTP requests will be needed to be sent. I'm assuming it'll be a GET to receive a cookie, and then one POST with the need parameters(Zipcode and Email address, along with the check of a terms button), although there may be another GET when first requesting a guest session.
As far as the request go, from my searches it looks like cURL is the way to go. Is this correct? -
2016-01-27 at 1:47 PM UTCIt's 7AM and i've been up all night, so I don't really want to type a bunch of code but fuck it.
By the way, if anyone wants to learn shell scripting and pen testing check outhttps://overthewire.org/wargames/bandit/
do not use ifconfig it is soon to be deprecated.
Highly recommended to use macchanger
run this, or macchanger as a cron job: http://www.unixgeeks.org/security/ne...ix/cron-1.html
[FONT=Trebuchet MS]1. IDGAF (I Don't Give A Fuck) what you do with my code*.[/FONT]
[FONT=Trebuchet MS]2. If you claim my code as your own, you will probably just be embarrassing yourself.[/FONT]
[FONT=Trebuchet MS]* As long as you don't use my code to make gay pronz[/FONT]
#!/bin/sh
# add me to cron job
#released under IDGAF licence
#1. IDGAF (I Don't Give A Fuck) what you do with my code*.
#2. If you claim my code as your own, you will probably just be embarrassing yourself.
#* As long as you don't use my code to make gay pronz.
if [ "$(id -u)" != "0" ]; then
echo "This script must be run as root nigga" 1>&2
exit 1
fi
interface="wlan0"
#uses IP as ifconfig is soon to be depricated
old_mac=$(ip link show wlan0 | awk '/link\/ether/ {print $2}')
new_mac=$(dd bs=1 count=5 if=/dev/random 2>/dev/null |hexdump -v -e '/1 "%02X-"';echo -n 00)
echo "($interface) Changing mac [$old_mac] to [$new_mac]\n"
# not the best
ip link set dev $interface down
ip link set dev $interface address $new_mac
ip link set dev $interface up
# better to use
# macchanger -r $interface # fully random mac
# macchanger -e $interface # changes device info, leaves vendor as is.
# macchanger -p $interfaxe # go back to hardware defined value
use curl or curl.
Write a program that does the website part of things, and just call macchanger and all will be good for spectraL -
2016-01-27 at 6:24 PM UTC
Here's what I have so far. I can spoof my MAC to a random address with this and I can return it back to the original(but it does require me to enter it in before hand).
#!/bin/bash
hexchars="0123456789ABCDEF"
rndMAC=
origMAC=00:00:00:11:23:11
function help() {
cat << EOF
Usage: $0 [args]
-h, --help - Print this help and exit
-r, --run - Spoof MAC every 55 minutes and connect/login to hotspot
-s, --spoof - Spoof MAC address to a randomly generated address
-u, --unspoof - Return spoofed MAC address to original MAC address
EOF
}
function genMac() {
end=$( for i in {1..10} ; do echo -n ${hexchars:$(( $RANDOM % 16 )):1} ; done | sed -e 's/\(..\)/:\1/g' )
rndMAC=00$end
}
function changeMac() {
MAC="$1"
service network-manager stop
ifconfig wlan0 down
ifconfig wlan0 hw ether $MAC
ifconfig wlan0 up
service network-manager start
}
function run() {
genMac
changeMac $rndMAC
#should automaticall connect due to previous connections
#login is next
#wait 55 minutes
run
echo "TODO"
}
if [ $UID -gt 0 ] ;then
die "RUN AS ROOT NIGGA"
fi
while [ $# -gt 0 ]
do
case "$1" in
"-h"|"--help")
help
die
;;
"-r"|"--run")
run
;;
"-s"|"--spoof")
genMac
changeMac $rndMAC
;;
"-u"|"--unspoof")
changeIface $origMAC
;;
*)
help
die
;;
esac
shift
done
I'll use BurpSuite when signing up for a new session and see what kind of HTTP requests will be needed to be sent. I'm assuming it'll be a GET to receive a cookie, and then one POST with the need parameters(Zipcode and Email address, along with the check of a terms button), although there may be another GET when first requesting a guest session.
As far as the request go, from my searches it looks like cURL is the way to go. Is this correct?
Yeah i'd use curl.
Also:It's 7AM and i've been up all night, so I don't really want to type a bunch of code but fuck it.
By the way, if anyone wants to learn shell scripting and pen testing check outhttps://overthewire.org/wargames/bandit/
do not use ifconfig it is soon to be deprecated.
Highly recommended to use macchanger
run this, or macchanger as a cron job: http://www.unixgeeks.org/security/ne...ix/cron-1.html
[FONT=Trebuchet MS]1. IDGAF (I Don't Give A Fuck) what you do with my code*.[/FONT]
[FONT=Trebuchet MS]2. If you claim my code as your own, you will probably just be embarrassing yourself.[/FONT]
[FONT=Trebuchet MS]* As long as you don't use my code to make gay pronz[/FONT]
#!/bin/sh
# add me to cron job
#released under IDGAF licence
#1. IDGAF (I Don't Give A Fuck) what you do with my code*.
#2. If you claim my code as your own, you will probably just be embarrassing yourself.
#* As long as you don't use my code to make gay pronz.
if [ "$(id -u)" != "0" ]; then
echo "This script must be run as root nigga" 1>&2
exit 1
fi
interface="wlan0"
#uses IP as ifconfig is soon to be depricated
old_mac=$(ip link show wlan0 | awk '/link\/ether/ {print $2}')
new_mac=$(dd bs=1 count=5 if=/dev/random 2>/dev/null |hexdump -v -e '/1 "%02X-"';echo -n 00)
echo "($interface) Changing mac [$old_mac] to [$new_mac]\n"
# not the best
ip link set dev $interface down
ip link set dev $interface address $new_mac
ip link set dev $interface up
# better to use
# macchanger -r $interface # fully random mac
# macchanger -e $interface # changes device info, leaves vendor as is.
# macchanger -p $interfaxe # go back to hardware defined value
use curl or curl.
Write a program that does the website part of things, and just call macchanger and all will be good for spectraL
I don't know who you are, but you should should stay, hang out with us, make scripts, pwn noobs that sort of thing. You strike me as a person who enjoyes those types of activities. -
2016-01-28 at 2:48 AM UTCAlright, I'll replace "ifconfig" with "ip link". I'm not going to use macchanger since the code I have, already spoofs my mac. I'll find some time to work on this and post my results.
-
2016-01-28 at 10:09 AM UTCifconfig has been on the "deprecated" list since like 2010? Nice post notreal
-
2016-01-28 at 12:48 PM UTCyou don't use psudorandom numbers to generate the new MAC, and rather than parsing ifconfig or ip for the hardware mac you 'manually' assign it.
You don't know how to use cron jobs.
I mean you were even going to use a proxy to outline the website, when you could view the source, use the devel tools in all modern browsers. You look at the html and recreate it in Python, C, Java, C++, C, Assembly, C, C++, Lua, C, C use C
Wrire the curl portion of the site, and use GNU macchanger like a real man
times like this i need to masturbate furiously
bahhh humbug -
2016-01-28 at 2:10 PM UTC
You don't know how to use cron jobs.
He could also just use the "at" command and have the script call the "at" command after every run ie.
#!/bin/bash
<change my mac>
at now + 55m /home/sbt/script.sh
and run this script the first time with "at now + 55m /home/sbt/script.sh" and it'll just call itself at the right time intervals infinitely. -
2016-01-28 at 6:28 PM UTCYou can also use a spoon to cut shit, but unless I'm in prison I prefer to use things for what there made for.
AT is used to schedule a task once, cron schedules a time for something to run in perpetuity. Use the right tools for the job, learn to see things like that, and become a better programmer.
I'm not tryin to be an ass, I just am one. Nigger shit. Do things the right way. Nigger shit.
Do it the right way, become a better programmer. Fail, suck at it, makes you a better coder. But learn the right way.
Nigger shit right here. Nigger shit. -
2016-01-28 at 11:10 PM UTC
I mean you were even going to use a proxy to outline the website, when you could view the source, use the devel tools in all modern browsers. You look at the html and recreate it in Python, C, Java, C++, C, Assembly, C, C++, Lua, C, C use C
I want to see what the full request looks like so I know what kind of request to send(specifically a possible cookie in the headers). Viewing the source wont show me the headers.
I've been using Burp Suite to get the POST/GET requests on a lot of different projects, and it's worked well.
Please let me know how I could recreate the request by viewing just the HTML.. -
2016-01-29 at 1:25 AM UTC
You can also use a spoon to cut shit, but unless I'm in prison I prefer to use things for what there made for.
AT is used to schedule a task once, cron schedules a time for something to run in perpetuity. Use the right tools for the job, learn to see things like that, and become a better programmer.
I'm not tryin to be an ass, I just am one. Nigger shit. Do things the right way. Nigger shit.
Do it the right way, become a better programmer. Fail, suck at it, makes you a better coder. But learn the right way.
Nigger shit right here. Nigger shit.
Cron schedules things things at absolute offsets ie. 3:45am erry monday. At can schedule things relatively ie. now + 23 minutes. If you don't know the exact time an event will happen (ie. mac change = captive portal log on), cron gives you an inefficient strategy. This is a very similar scenario to polling vs. long-polling - long-polling is always more efficient.I want to see what the full request looks like so I know what kind of request to send(specifically a possible cookie in the headers). Viewing the source wont show me the headers.
I've been using Burp Suite to get the POST/GET requests on a lot of different projects, and it's worked well.
Please let me know how I could recreate the request by viewing just the HTML..
Yeah, it's possible - you check out the html to find the form and gather the info from there - the "action" attribute will tell you the url that the is submitted to, the method will tell you the method (surprise!) and the html inputs fields will tell you the key value pairs to send. The method attribute of the form tag will also tell you whether the key value pairs should be appended to the url as in a get request or in the request body as in the post request. But, as you say, this won't give you the headers, so if you need the cookie - you're fucked. Add to that the prevalence of javascript and you might also be missing out on other headers/modifications that are happening that are not reflected in the html document.