
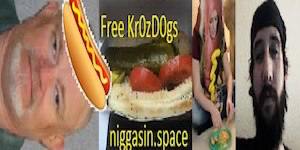
User Controls
Grabbing Crypto Addresses with regex. And more.
-
2021-12-20 at 3:02 PM UTCPreamble
I'd like to use this thread as a bit of a resources to post regex that may be useful in filtering and identifying crypto addresses and other strings with regards to financial information.
regex
py_dict= {'legacy_btc': '^[13][a-km-zA-HJ-NP-Z1-9]{25,34}$',
'segwit_btc': '^(bc1|[13])[a-zA-HJ-NP-Z0-9]{25,39}$',
'xmr': '4[0-9AB][123456789ABCDEFGHJKLMNPQRSTUVWXYZabcdefghijkmnopqrstuvwxyz]{93}',
'eth': '^0x[a-fA-F0-9]{40}$',
'lite': '^[LM3][a-km-zA-HJ-NP-Z1-9]{26,33}$',
'dash': '^X[1-9A-HJ-NP-Za-km-z]{33}$',
'ripple': '^r[0-9a-zA-Z]{24,34}$',
'doge': '^D{1}[5-9A-HJ-NP-U]{1}[1-9A-HJ-NP-Za-km-z]{32}$'}
This is a Python dictionary object that has regex for the crypto coins you can see defined. Dictionaries like this are handy for a number of reasons not least of all since it allows you to easily read/write JSON files. Therefore when it comes to any program that needs the ability to recognize certain strings as valid crypto addresses you can easily write a config file in JSON format to specify the types of coins you're after.
You could also have a dictionary like this in your malware, say a keylogger, so it can automatically recognize/grab/copy/replace any strings like this by comparing the keystrokes to your regexes. If you're gonna make a python keylogger though i do suggest you sue the Ctypes lib, and create a proper keymap, like you would in a C or C++ based malware instead of relying on PyHook and such to hook the keyboard. On that note handling registry operations in Python with Ctypes is definitely the way to go as well.
Below is a small sample of regex, that should work with C++.
regex bitpat{ "^(bc1|[13])[a-zA-HJ-NP-Z0-9]{25,39}$" };
regex litpat{ "^[LM3][a-km-zA-HJ-NP-Z1-9]{26,33}$" };
regex monpat{ "^4([0-9]|[A-B])(.){93}" };
regex ethpat{ "^0x[a-fA-F0-9]{40}$" };
I had a bunch more regexes for CC info, important strings to do with banking such as IBAN and SEPA, but i got those on a box, that is currently offline and in need of some repairs i can't be assed to get the HDD and look for the specific files in question.
This is just a small sample size, please feel free to add some more.
Moar
Related to this, if you'e in the business of hijacking API keys for payment processors the following might be for you. not exactly regex but ways to verify the API tokens/secrets you may come across.
API verify and info
Paypal
Paypal client id and secret key
curl -v https://api.sandbox.paypal.com/v1/oauth2/token \
-H "Accept: application/json" \
-H "Accept-Language: en_US" \
-u "client_id:secret" \
-d "grant_type=client_credentials"
The access token can be further used to extract data from the PayPal API. More info
This can be verified using:
curl -v -X GET "https://api.sandbox.paypal.com/v1/identity/oauth2/userinfo?schema=paypalv1.1" -H "Content-Type: application/json" -H "Authorization: Bearer [ACCESS_TOKEN]"
Stripe
Stripe Live Token
curl https://api.stripe.com/v1/charges -u token_here:
Keep the colon at the end of the token to prevent cURL from requesting a password.
The token is always in the following format: `sk_live_24charshere`, where the `24charshere` part contains 24 characters from `a-z A-Z 0-9`. There is also a test key, which starts with `sk_test`, but this key is worthless since it is only used for testing purposes and most likely doesn't contain any sensitive information. The live key, on the other hand, can be used to extract/retrieve a lot of info — ranging from charges to the complete product list.
Keep in mind that you will never be able to get the full credit card information since Stripe only gives you the last 4 digits.
More info/complete documentation https://stripe.com/docs/api/authentication.
Razorpay
Razorpay API key and Secret key
This can be verified using:
curl -u <YOUR_KEY_ID>:<YOUR_KEY_SECRET> \
https://api.razorpay.com/v1/payments
Anyway figured i'd post it here to get a good list going and provide those that are/were unaware, with this information for your enjoyment. Got anything interesting to add? Please feel free to do so.The following users say it would be alright if the author of this post didn't die in a fire! -
2021-12-20 at 11:25 PM UTCyou should be able to shorten xmr code to:
'xmr': '^4[0-9AB][1-9A-HJ-NP-Za-km-z]{93}$',
in your dict list, correct me if this causes an error, as i did not test in a live env. -
2021-12-21 at 4:18 AM UTCit always throws me how different languages use slightly different operators
I wish regex was available in a lot more apps like web searches and the like; just give each search a maximum number of clock cycles depending on current load
re: keylogging, who actually types wallet addresses by hand -
2021-12-22 at 4:07 AM UTC
Originally posted by aldra it always throws me how different languages use slightly different operators
I wish regex was available in a lot more apps like web searches and the like; just give each search a maximum number of clock cycles depending on current load
re: keylogging, who actually types wallet addresses by hand
If your keylogger doesn't capture shit that ends up in 'the clipboard' it's a sub par implementation. And yeah, i hate regex, which is why Maddie is probably right about the changes to the one she mentioned.
Also if you wanna do web searches with regex use Selenium and your webdriver + supported lang of choice. And of course while not really regex google does have advanced search operators. -
2022-01-05 at 1:12 AM UTCI haveat least one I wrote that list CC numbers using the Luhn algorithum. It's in Java though(I made an Android app as well but that one is for something else...). Not sure if thats allowed here.
-
2022-01-06 at 1:54 AM UTC
-
2022-01-07 at 4:27 AM UTC
package com.lanny.things;
import java.io.BufferedWriter;
import java.io.File;
import java.io.FileWriter;
import java.io.IOException;
import java.util.ArrayList;
public class Nir {
public static ArrayList<int[]> numberSet;
public static String number1 = "4343404100000000";
public static String number2 = "4343404199999999";
public static String numberToCheck = "4343404105441234";
public static int[] checkNumber;
public static boolean check = false;
public static boolean writeToFile = false;
public static void main(String[] args) throws Exception {
if (!check){
numberSet = new ArrayList<int[]>();
int[] a = new int[16], b = new int[16];
for (int i = 15; i > -1; i--){
a[i] = number1.charAt(i) - 48;
b[i] = number2.charAt(i) - 48;
}
createList(a, b);
for (int n = 0; n < numberSet.size(); n++){
int[] temp = numberSet.get(n);
for (int i = 0; i < 16; i++){
System.out.print(temp[i]);
}
System.out.println();
}
} else {
checkNumber = new int[16];
for (int i = 15; i > -1; i--){
checkNumber[i] = numberToCheck.charAt(i) - 48;
}
System.out.println(isValid(checkNumber)?"Valid":"Not Valid");
}
}
public static void createList(int[] range1, int[] range2){
while(!equals(range1, range2)){
if (isValid(range1)){
int[] temp = new int[16];
for (int i = 0; i < 16; i++){
temp[i] = range1[i];
}
numberSet.add(temp);
if (writeToFile){
try {
File file = new File("/home/lanny/Desktop/NumberList");
BufferedWriter output = new BufferedWriter(new FileWriter(file, true));
StringBuilder sb = new StringBuilder();
for (int i = 0; i < 16; i++){
sb.append(temp[i]);
}
output.write(sb.toString() + "\n");
output.close();
} catch (IOException e) {e.printStackTrace();}
}
}
range1 = increment(range1);
}
}
public static boolean isValid(int[] n){
int total = 0;
for (int i = 15; i > -1; i--){
if (i % 2 != 0){
total = total + n[i];
} else {
Integer temp = n[i] + n[i];
int tempt = 0;
for (int x = 0; x < temp.toString().length(); x++){
tempt = tempt + Integer.parseInt(String.valueOf(temp.toString().charAt(x)));
}
total = total + tempt;
}
}
return (total % 10 == 0);
}
public static int[] increment(int[] i){
int[] returner = i;
boolean finished = false;
int s = 15;
while (!finished){
if (i[s] != 9){
returner[s]++;
finished = true;
} else {
returner[s] = 0;
s--;
}
}
return returner;
}
public static boolean equals(int[] range1, int[] range2){
for (int i = 0; i < 16; i++){
if (range1[i] != range2[i]) return false;
}
return true;
}
}The following users say it would be alright if the author of this post didn't die in a fire! -
2022-01-07 at 4:11 PM UTCThank you Migh. Very cool.
-
2022-01-09 at 1:36 AM UTC
Originally posted by Migh I haveat least one I wrote that list CC numbers using the Luhn algorithum. It's in Java though(I made an Android app as well but that one is for something else…). Not sure if thats allowed here.
i wrote something similar to this but in python2 a few years ago.
nice script btw